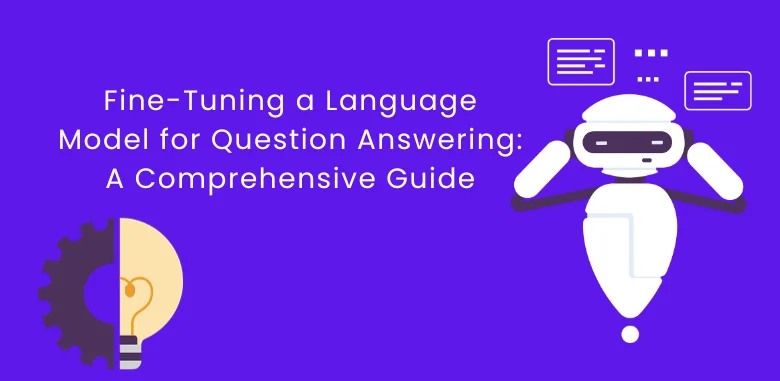
In recent years, Question Answering (QA) systems have gained significant prominence in natural language processing. They are essential for tasks like information retrieval, virtual assistants, and much more. To build an effective QA system, fine-tuning a Large Language Model (LLM) is often the key. In this comprehensive guide,you will unravel the process of fine-tuning a language model for question answering, providing code examples and explanations along the way.
Why Fine-Tuning?
Fine-tuning a pre-trained language model offers several advantages:
1. Transfer Learning
Leveraging pre-trained models allows you to benefit from the vast amount of knowledge they’ve acquired during their initial training. These models have been trained on massive text corpora, learning linguistic patterns and world knowledge that can be invaluable for a wide range of NLP tasks, including QA.
2. Cost-Efficiency
Fine-tuning is typically faster and requires less data than training a model from scratch. Pre-trained models serve as a good point to get started, reducing the computational resources and time needed for training.
3. Performance
Fine-tuned models can achieve state-of-the-art results on a wide range of NLP tasks, including QA. By adapting a pre-trained model to a specific task or domain, you can unlock its full potential.
Prerequisites
Before learning about the fine-tuning process, ensure you have the following:
Hardware: A machine with suitable hardware, such as a GPU, can significantly accelerate the training process.
Python: Python is the primary programming language used in NLP. Install Python (preferably Python 3.x) and essential libraries like PyTorch or TensorFlow.
Framework Knowledge: Basic understanding of PyTorch or TensorFlow is recommended, as we’ll use these frameworks for fine-tuning.
Now, let’s explore the step-by-step process of fine-tuning a language model for question answering.
Step 1: Choose a Pre-trained Model
Start by selecting a pre-trained language model. Several models are well-suited for QA tasks, including BERT, RoBERTa, T5 , LLAMA and GPT-2. Let’s briefly compare them:
BERT (Bidirectional Encoder Representations from Transformers): BERT is known for its bidirectional context understanding, making it strong in capturing context. However, it may be computationally intensive, especially for larger models.
RoBERTa: RoBERTa is a variant of BERT with improved training techniques, often leading to better performance. It refines pre-training strategies and offers robust results.
T5 (Text-to-Text Transfer Transformer): T5 is another powerful pre-trained language model. What sets T5 apart is its text-to-text framework, where it frames many NLP tasks, including question answering, as a text-to-text problem. It has shown impressive results across various natural language understanding tasks and is known for its simplicity and effectiveness in fine-tuning for specific tasks.
GPT-2 (Generative Pre-trained Transformer 2): GPT-2 is an earlier version of GPT-3. While it’s not as large as GPT-3, it’s still a substantial model with impressive text generation capabilities. It requires fewer computational resources to train and fine-tune, making it a more accessible choice for many projects.
GPT-3: GPT-3 is indeed a very large Language Model and is known for its remarkable language generation abilities. However, it’s important to note that fine-tuning GPT-3 for specific tasks, including QA, can be computationally intensive and may require substantial resources. Due to its size and complexity, it’s often recommended to start with smaller models like GPT-2 or T5 if you’re working on projects with limited computational resources.
For this guide, we’ll use the Hugging Face Transformers library, a popular choice for NLP tasks, to fine-tune a BERT-based model. Let’s load the model and tokenizer.
from transofrmers import BertForQuestionAnswering, BertTokenizer
model_name = "bert-base-uncased"
model = BertForQuestionAnswering.from_pretrained(model_name)
tokenizer = BertTokenizer.from_pretrained(model_name)
Step 2: Prepare Your QA Dataset
To fine-tune a QA model, you need a labeled QA dataset. While you can create your own, popular choices include the Stanford Question Answering Dataset (SQuAD) or a domain-specific dataset. Here’s an overview of the SQuAD dataset:
Stanford Question Answering Dataset (SQuAD)
SQuAD is widely used for QA tasks. It contains passages from various sources, each with questions and corresponding answers. This dataset is well-structured and labeled, making it a suitable choice for fine-tuning.
If you decide to create your own dataset, adhere to these guidelines:
High-Quality Passages: Ensure your passages are high-quality, diverse, and relevant to the questions you intend to answer.
Clear Questions and Accurate Answers: Craft clear, concise questions and provide accurate answers. Ambiguity in questions or answers can lead to model confusion.
Labeling: Utilize crowd-sourcing platforms or expert annotators for labeling. Maintain a rigorous labeling process to ensure data quality. You might even use a language model to generate labeled question answers for you!
Consistent Format: Maintain a consistent format for your dataset, such as question-context-answer triplets. This consistency simplifies data preprocessing.
Step 3: Data Preprocessing
Data preprocessing is a crucial step in fine-tuning a language model for QA. It involves three primary components: tokenization, encoding, and batching.
Tokenizing
Tokenization is the process of breaking text into smaller units, typically words or subwords. In NLP, words are converted into tokens, which are numerical representations used by the model. Tokenization helps the model understand the input text.. However, it’s important to note that tokenization isn’t a one-size-fits-all process; different use cases may require different tokenization techniques.
from transformers import BertTokenizer
tokenizer = BertTokenizer.from_pretrained(model_name)
text = "This is an example sentence."
tokens = tokenizer(text, return_tensors="pt")
In this example, the text is tokenized using the BERT tokenizer. The return_tensors parameter specifies that the tokens should be returned as PyTorch tensors.
Encoding
Encoding is the process of converting tokens into numerical values that can be fed into the model. It maps each token to its corresponding index in the model’s vocabulary.
Input_ids = tokens["input_ids"]
The input_ids represent the numerical input to the model, where each value corresponds to a token.
Batching
Batching involves grouping multiple encoded sequences together into a single input batch. This allows for parallel processing and efficient use of resources during training.
from torch.utils.data import DataLoader
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
In this code snippet, a DataLoader is created to handle batching. The batch_size determines how many sequences are processed together in each batch, and shuffle=True shuffles the data to introduce randomness into training.
Step 4: Training
With your data prepared, it’s time to fine-tune the model. You’ll need to define training parameters and monitor the loss during training. Let’s set up the training process:
from transformers import Trainer, Training Arguments
training_args = TrainingArguments(
output_dir="./qa_finetuned_model"<
per_device_train_batch_size=8,
num_train_epochs=2,
evaluation_strategy="steps",
eval_steps=1000,
save_steps=1000,
)
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=dataset["train"],
eval_dataset=dataset["validation"],
)
Here’s a breakdown of the key training parameters:
output_dir: This is the directory where model checkpoints and outputs will be saved.
per_device_train_batch_size: It specifies the batch size used during training. Larger batch sizes may require more GPU memory.
num_train_epochs: This parameter determines the number of times the training data is passed through the model, affecting the total training time.
evaluation_strategy: Specifies when to evaluate the model during training. In this case, we use “steps,” which means evaluating every eval_steps training steps.
eval_steps: Specifies how often (in training steps) to perform evaluation. Frequent evaluation helps track the model’s progress.
save_steps: Determines how often (in training steps) to save model checkpoints. Checkpoints are essential for resuming training or using the model for inference.
Now, you can start training the model:
trainer.train()
During training, the model will iterate through your dataset, updating its weights to minimize the loss.
Step 5: Evaluation
After training, it’s crucial to evaluate the model’s performance on the validation set to assess its ability to answer questions accurately. The evaluation process involves using specific metrics to measure the model’s performance. Common metrics for QA include BLEU score and the ROGUE score.
Understanding Evaluation Metrics
BLEU Score
BLEU measures the similarity between model-generated and reference answers by considering shared word sequences, allowing for partial matches and variations in phrasing.
ROUGE Score
ROUGE evaluates overlap between model-generated and reference answers based on n-grams and word sequences, accommodating differences in language and structure.
The choice of metrics depends on your specific QA task. In most cases, higher BLEU and ROUGE scores indicate better performance. A high score suggests that the model’s generated answers are more similar to the reference answers, which is typically desired, but the threshold for what constitutes a “good” model can vary depending on the complexity of your dataset and task.
You can perform evaluation with the following code:
results = trainer.evaluate()
print(results)
The results object will contain various metrics, including EM and F1 scores, giving you insights into the model’s performance.
Step 6: Inference
Once your model is fine-tuned and evaluated, you can use it for real-world QA tasks. Here’s how you can perform inference with your fine-tuned model:
question = "What is the capital of France?"
context = "Paris is the capital of France."
input = tokenizer(question, context, return_tensors="pt")
start_logits, end_logits = model(**inputs)
start_idx = torch.argmax(start_logits)
end_idx = torch.argmax(end_logits)
answer_tokens = inputs["input_ids"][0][start_idx:end_idx+1]
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(answer_tokens))
print(answer)
In this code snippet, we provide a question and context to the model. The model computes start and end logits, indicating the positions of the answer span. We then extract the answer tokens and convert them back into a human-readable answer string.
Suggestions and Best Practices
Fine-tuning a language model for question answering is a complex process that requires experimentation and careful consideration of various factors. Here are some suggestions and best practices to help you achieve the best results:
Experiment with Hyperparameters: Fine-tuning performance heavily depends on hyperparameters like batch size, learning rate, and training duration. Experiment with different values and techniques to optimize results for your specific task.
Use Domain-Specific Data: If your QA task is domain-specific (e.g., medical or legal), consider fine-tuning on domain-specific data. Models fine-tuned on domain-specific data often perform better in specialized tasks.
Regularly Save Checkpoints: Save model checkpoints during training at regular intervals. This practice allows you to resume training from the last saved checkpoint if needed and helps prevent data loss in case of unexpected interruptions.
Monitor Training Progress: Keep a close eye on the training loss and validation metrics during training. Early detection of issues or suboptimal performance can save time and resources.
Data Augmentation: Consider data augmentation techniques to diversify your training data. Techniques such as paraphrasing questions or providing additional context can help improve model generalization.
Regular Fine-Tuning: Language models evolve over time, and new pre-trained models are released. Regularly re-evaluate and fine-tune your models to keep up with the latest advancements in NLP.
Fine-Tuning Considerations and Alternatives
Before we wrap up, it’s crucial to touch on some advanced techniques and considerations in the process of fine-tuning language models. While fine-tuning can be a powerful tool for adapting pre-trained models to specific tasks, it’s not without its challenges. Two significant concerns are catastrophic forgetting and overfitting to the new data.
Catastrophic Forgetting
Catastrophic forgetting occurs when a language model is fine-tuned on new data, and in the process, it forgets its previous generalization and knowledge. This phenomenon can limit the model’s ability to perform well on a range of tasks if it is continually updated with new information. Users must be cautious when deploying fine-tuned models in dynamic environments.
To address this issue, researchers have been exploring techniques such as Progressive and Elastic Few-shot Transfer (PEFT) and Learning without Forgetting (LORA). PEFT aims to make models more adaptable by allowing them to learn new tasks without severely affecting their performance on previously learned ones. LORA, on the other hand, focuses on mitigating catastrophic forgetting by preserving knowledge while adapting to new data.
Soft Prompting
Another promising avenue is Soft Prompting. It’s a technique that provides a more controlled way to fine-tune models. Instead of traditional prompt engineering, where prompts are hard-coded, Soft Prompting allows models to generate prompts dynamically based on the task or context. This approach offers flexibility and adaptability, making it easier to fine-tune models effectively.
Considering Alternatives
As an alternative to fine-tuning, users may also consider a few-shot learning approach, where the model is trained to generalize from a small number of examples. Few-shot learning can be a more robust solution for certain tasks, as it doesn’t suffer from catastrophic forgetting to the same extent.
Here is the article on Predictive Analytics in Health Niche.
Conclusion
In conclusion, fine-tuning a language model for question answering is a powerful approach to build robust QA systems. It leverages the knowledge and capabilities of pre-trained models, making it accessible even for those with limited resources. Follow the steps outlined in this guide, experiment, and continuously improve your QA system to meet your specific needs. In the ever-evolving field of NLP, staying informed about the latest techniques, staying flexible and adaptive is the key to unlocking the full potential of these remarkable language models in the pursuit of more intelligent, conversational AI and NLP applications. Happy fine-tuning!
Author
Team Solutyics is a dynamic group of Analytics and AI specialists who bring together a rich mix of expertise. Their combined insights ensure that readers gain a deeper understanding of practical applications of Analytics and AI.